Top-up by Apple Pay allows your customers to top-up IBAN opened at ConnectPay by Apple Pay’s digital wallet.
Prerequisites:
- Before starting your integration, you’ll need to prepare your environment: you must place the domain validation file on your server at: https://domain/.well-known/apple-developer-merchantid-domain-association. Without this file, it will not be possible to use Apple Pay on your domain. ConnectPay engineers will provide you that file (it’s different for non-prod and production environment).
- Also, you’ll need to white-list Apple IP’s and domain names for merchant validation in production and testing. You can find the list here. Do not allow your server to access any other URLs for merchant validation.
- Apple merchant ID, domain Name – for account funding transactions we will share specific Apple Pay merchant ID and domain Name, you don’t need to create it in Apple Developer portal. These parameters are different for non-prod and production environment.
Apple Pay top-up payments are settled instantly, you don’t need to wait as it is for commercial transactions.
Flow:
Integration can be split in two parts – merchant validation together with session creation and payment initiation.
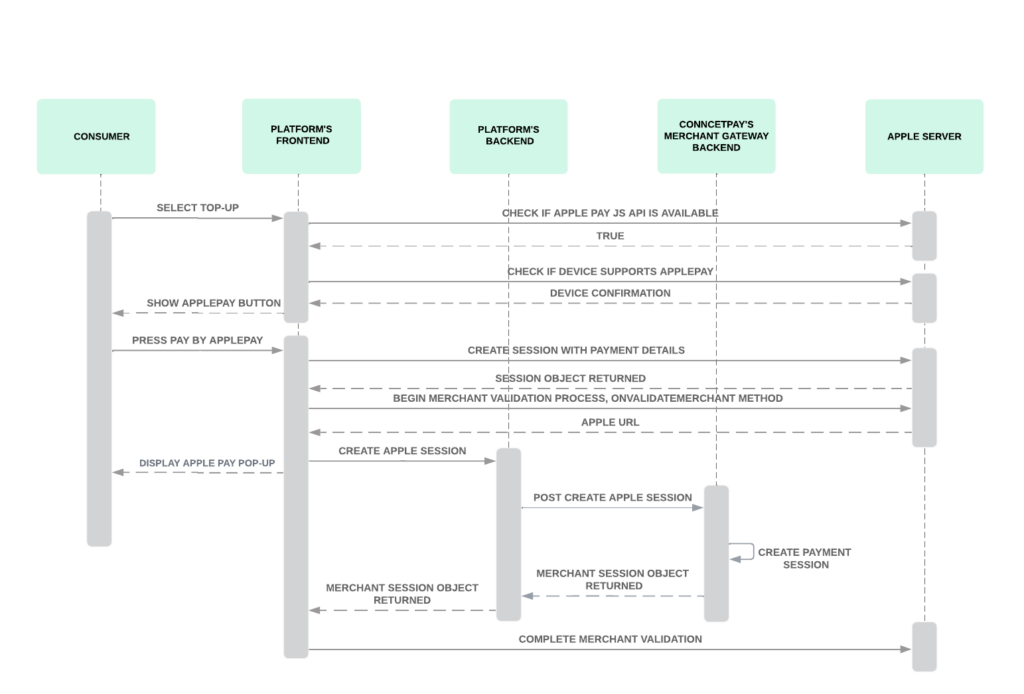
- When your end-user selects to top-up by Apple Pay, you need to check if they are using Safari browser by checking if window.ApplePaySession class exist.
- After that, you’ll need to check if device supports it. This can be done by calling Apple canMakePayments method. If you receive
true
value, then device supports making payments with Apple Pay and you can show Apple button on your frontend. It doesn’t verify whether or not the user has any provisioned cards in Wallet. If you want to show Apple button only for those users who have active cards added to the Wallet, you can use canMakePaymentsWithActiveCard method. If returned true, then device supports Apple Pay and there is at least one active card in Wallet that is qualified for payments on the web.
if(window['ApplePaySession'] && ApplePaySession.canMakePayments("pass merchant id here"))
- You’ll need to style Apple Pay button based on Apple’s requirements. Styling done against requirements might bring blocking from Apple after some time.
- After you decide to show Apple Pay button and user selects to Pay, you’ll need to create a session with Apple by initiating Apple Pay session. For version parameter follow Apple’s guidelines, for payment request parameters, use:
countryCode
– LT;currencyCode
– selected currency, for now EUR only;supportedNetworks
– visa, mastercardmerchantCapabilities
– supports3DSlabel
– your Platform name or what you want to show on Apple pop-up;amount
– amount from user’s selection.
var request = { countryCode: 'LT', currencyCode: 'EUR', supportedNetworks: ['visa', 'masterCard'], merchantCapabilities: ['supports3DS'], total: { label: 'Your Merchant Name', amount: '10.00' }, } var session = new ApplePaySession(3, request);
- After the session is created, call its begin method to show the payment sheet. When this method is called, the payment sheet is presented and the merchant validation process is initiated. Then use
onvalidatemerchant
function to start validation process.
const request = {
countryCode: 'LT',
currencyCode: 'EUR',
supportedNetworks: ['visa', 'masterCard'],
merchantCapabilities: ['supports3DS'],
total: { label: 'ConnectPay', amount },
}
const session = new ApplePaySession(3, request);
session.begin();
session.onvalidatemerchant = (event) => {
const { validationURL } = event;
// do the validation here
// after receiving the session object from back end call
session.completeMerchantValidation(" by passing the object here");
};
- After Apple validates the session and provides session URL, send this URL together with merchant identifier, domain name and display name received from us using Create Apple Pay Session API .
- We will complete merchant validation with Apple, create a session and return you merchant session object. Don’t modify this object, you’ll need to use it for payment authorization flow.
- Pass merchant session object received from us to Apple using completeMerchantValidation method to enable the user to authorize a transaction. The merchant session object expires 5 minutes after it is created.
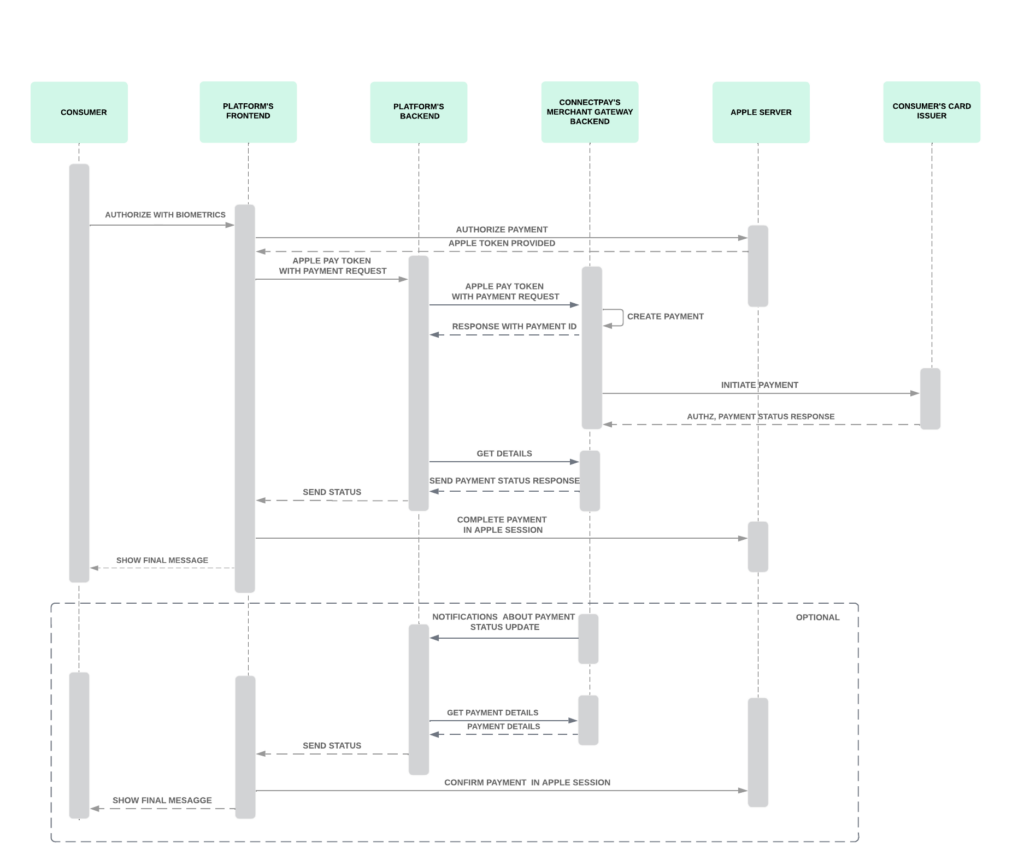
- When your session is validated and created at Apple side, user can now authorize a payment. When user authorizes a payment, onpaymentauthorized function is called. The event parameter contains the payment attribute. The
onpaymentauthorized
function must complete the payment and respond by callingcompletePayment
before the 30 second timeout, after which a message appears stating that the payment couldn’t be completed.
session.onpaymentauthorized = (event)=> {
const token = event.payment.token;
// handling the payment authorization
}
- Take Apple token from
onpaymentauthorized
function and send it to us using Initiate Apple Pay top up API. We will create a payment and send it to your customer’s issuer. - For receiving payment status you have 2 options: calling our Get payment details API using
paymentId
provided in Initiate Apple Pay top up API response or receiving payment status from Notifications (you need to subscribe notifications to do that). - After you receive terminal payment status from us –
Failed
orApproved
/Settled
, you need to complete payment on Apple side using completePayment method. Until this method is called Apple will load spinner on UI blocking user from further actions or time out it after 30 seconds claiming payment couldn’t be completed. If payment status isPendingProvider
orProcessing
, retry calling Get payment details API until you receive terminal status. - After payment is completed on Apple, you can decide what to do with user journey – show success/failure message or redirect to other page.
Tip! Before payment authorization flow, Apple session time out is 1 hour (time frame when user can select their card), if your session is shorter, you can handle it by calling abort function on your session time-out event. If you don’t align Apple session with your session time, after your session is expired, your user will be blocked by Apple pop-up.